Introduction :
The calculation of area and perimeter of geometrical shapes is an important and fundamental mathematical operation. That operation is used in various fields like engineering, architecture, computer graphics etc. C language is a powerful and popular programming language used for simple and complex mathematical calculations.
In this article, I shall show you how to calculate the area and perimeter of various geometrical shapes using the C programming language. The geometrical shapes are rectangle, square, circle, triangle, parallelogram, rhombus, trapezium, equilateral triangle, right-angled triangle and isosceles triangle
In this article, I shall demonstrate the formulas required for calculating the area and perimeter of different geometrical shapes. And also write specific code for each shape to implement these formulas in C programming language. To run the following program, I use VS Code. If you want to install VS Code in your pc follow this link.
- Calculate the area and perimeter of rectangle
- Calculate the area and perimeter of square
- Calculate the area and perimeter of circle
- Calculate the area and perimeter of triangle
- Calculate the area and perimeter of parallelogram
- Calculate the area and perimeter of rhombus
- Calculate the area and perimeter of trapezium
- Calculate the area and perimeter of equilateral triangle
- Calculate the area and perimeter of right-angled triangle
- Calculate the area and perimeter of isosceles triangle
What is Area and Perimeter :
Before seeing the following program of calculating the area and perimeter of shapes, you have to understand basic concepts of area and perimeter.
Area is a mathematical concept that measure of the surface covered by a two-dimensional shape. The unit of area is typically measured in square units such as square meters, square feet, or square inches.
Perimeter is a mathematical concept that measure the total length of the boundary of a two-dimensional shape. The unit of perimeter typically measured in linear units, such as meters, centimeters, or feet.
Calculate the area and perimeter of rectangle by C programming language :
In this program, I shall show you how to calculate the area and perimeter of a rectangle using the C programming language. Quadrilateral with four right angles and opposite sides are parallel and equal in length is called rectangle.
For calculating the area and perimeter of a rectangle, first you need to know its length and width. The following formula used for calculating the area of a rectangle.
area = length x width
The following formula used for calculating the perimeter of a rectangle.
perimeter = 2 x (length + width)
explanation of the program :
In this program, I first declare four floating variables “length”, “width”, “area” and “perimeter”. Then using printf() function ask the user to enter the length and width of the rectangle. Using scanf() function stores the length and width of the rectangle in “length” and “width” variable respectively.
Then calculate the area and perimeter of the rectangle and store in “area” and “perimeter” variables respectively. Finally, display the output using the format specifier %.2f to display the area and perimeter with two decimal places in printf() function. You can copy the below code of calculating the area and perimeter of rectangle by C programming language and run on your pc.
/* Developd by Puskar Jasu*/
#include <stdio.h>
int main() {
float length, width, area, perimeter;
printf("Enter the length of the rectangle: ");
scanf("%f", &length);
printf("Enter the width of the rectangle: ");
scanf("%f", &width);
area = length * width;
perimeter = 2 * (length + width);
printf("The area of the rectangle is: %.2f\n", area);
printf("The perimeter of the rectangle is: %.2f\n", perimeter);
return 0;
}
Output:
After running the above code you can see the output of calculating the area and perimeter of rectangle by C programming language.

Calculate the area and perimeter of square by C programming language :
In this program, I shall show you how to calculate the area and perimeter of a square using the C programming language. A square is a geometric shape with four equal sides and angles.
To calculate the area and perimeter of a square, you have to know the length of its side. Formula for calculating the area of a square given below.
area = side x side
Formula for calculating perimeter of a square given below.
perimeter = 4 x side
explanation of the program :
In this program, I first declare three floating variables such as “side”, “area”, and “perimeter”. Then use the printf() function for asking the user to enter the length of one side of the square and use scanf() function to store it in the “side” variable.
Next, calculate the area and perimeter of the square and store the results in the “area” and “perimeter” variables respectively. Finally, use the printf function to print the values of area and perimeter to the screen.
Here, I use the format specifier %.2f to display the area and perimeter with two decimal places in printf() function. You can copy the below code of calculating the area and perimeter of square by C programming language and run on your pc.
/* Developd by Puskar Jasu*/
#include <stdio.h>
int main()
{
float side, area, perimeter;
printf("Enter the length of the side of the square: ");
scanf("%f", &side);
area = side * side;
perimeter = 4 * side;
printf("Area of square = %.2f\n", area);
printf("Perimeter of square = %.2f\n", perimeter);
return 0;
}
Output:
After running the above code you can see the output of calculating the area and perimeter of square by C programming language.

Calculate the area and perimeter of circle by C programming language :
In this program, I shall show you how to calculate the area and perimeter of a circle using the C programming language. A circle is a rounded shape that is formed by a set of points on a plane that are equidistant from a given point called the center.
To calculate the area and perimeter of a circle, you have to know its radius. The distance between the center of the circle and any point on the circumference of the circle is known as the radius of the circle.
The following formula is used for calculating the area of a circle.
area = π x (radius)2
The following formula is used for calculating the perimeter of a circle.
perimeter = 2 x π x radius
In the above formulas, π (pi) is a mathematical constant that is approximately equal to 3.14159.
explanation of the program :
In this program, first declare three float variables name as “radius”, “area”, and “perimeter” and also declare a constant variable “pi” and set its value to 3.14159. Then use the printf() function for asking the user to enter the radius of the circle. Using the scanf() function, store the value of the radius of the circle in “radius” variable.
Next, calculate the area and perimeter of the circle and store the result in the “area” and “perimeter” variable respectively. Finally, by the printf() function display the calculated area and perimeter of the circle on the screen using format specifier %.2f for display with two decimal places.
Now copy the below code for calculating the area and perimeter of circle by C programming language and run on your pc.
/* Developd by Puskar Jasu*/
#include <stdio.h>
int main()
{
float radius, area, perimeter;
const float pi = 3.14159;
printf("Enter the radius of the circle: ");
scanf("%f", &radius);
area = pi * radius * radius;
perimeter = 2 * pi * radius;
printf("Area of the circle = %.2f\n", area);
printf("Perimeter of the circle = %.2f\n", perimeter);
return 0;
}
Output:
After running the above code you can see the following image as output of calculating the area and perimeter of circle in C programming language.

Calculate the area and perimeter of triangle by C programming language :
In this program, I shall show you how to calculate the area and perimeter of a triangle using the C programming language. A triangle is a geometrical shape with three sides and angles. The sum of the angles in a triangle is always 180 degrees. To calculate the area and perimeter of a triangle, you have to know the length of its three sides.
Note that, using the following formula and program you can calculate the area and perimeter of scalene triangle by C programming language. A triangle with no equal sides and no equal angles is called scalene triangle.
There are different formulas to calculate the area of a triangle depending upon the type of triangle. The most general formula for calculating the area is Heron’s formula.
area = √(semiperimeter x (semiperimeter-side1) x (semiperimeter-side2) x (semiperimeter-side3))
Where side1, side2, side3 is the length of the triangle and semiperimeter of the triangle calculated by the following formula.
semiperimeter = (side1+side2+side3)/2
To calculate the perimeter of a triangle, simply add the lengths of its three sides.
perimeter = side1+side2+side3
explanation of the program :
In this program, first declare six float variables such as “side1”, “side2”, “side3”, “perimeter”, “area”, “semiperimeter”. Using the printf() function asked the user to enter the lengths of the three sides of the triangle. By the scanf() function, store the length of triangle in side1, side2 and side3 variables.
Next, calculate the perimeter, semiperimeter and the area of the triangle and store in “perimeter”, “semiperimeter” and “area” variables. After that using the printf() function displays the calculated area and perimeter of the triangle to the screen. Here format specifier %.2f used in printf() function to display the result with two decimal places. Now copy below code and run on your pc.
/* Developd by Puskar Jasu*/
#include <stdio.h>
#include <math.h>
int main()
{
float side1, side2, side3, perimeter, area, semiperimeter;
printf("Enter the length of the first side of the triangle: ");
scanf("%f", &side1);
printf("Enter the length of the second side of the triangle: ");
scanf("%f", &side2);
printf("Enter the length of the third side of the triangle: ");
scanf("%f", &side3);
perimeter = side1 + side2 + side3;
semiperimeter = perimeter / 2;
area = sqrt(semiperimeter * (semiperimeter - side1) * (semiperimeter - side2) * (semiperimeter - side3));
printf("Area of the triangle = %.2f\n", area);
printf("Perimeter of the triangle = %.2f\n", perimeter);
return 0;
}
Output:
You can see the following image as output of calculating the area and perimeter of triangle by C programming language.

Calculate the area and perimeter of parallelogram by C programming language :
In this program, I shall show you how to calculate the area and perimeter of a parallelogram using the C programming language. Quadrilateral with opposite sides parallel to each other is called parallelogram. To calculate the area and perimeter of a parallelogram, you need to know the length of its sides and the height.
The area of a parallelogram can be calculated by multiplying the base length by the height of the parallelogram. The following formula is used for calculating the area of a parallelogram.
Area = base x height
Where base is the length of one of the parallel sides and height is the distance between base and its parallel side.
The perimeter of a parallelogram is the sum of the lengths of all four sides. The following formula is used for calculating the perimeter of the parallelogram.
Perimeter = 2 x (length + width)
Where length and width are value of sides of a parallelogram.
explanation of the program :
In the program, you have to declare the required float variables such as “base”, “height”, “length” and “width”, “area”, and “perimeter”. Then, ask the user for entering values of base, height, length and width of a parallelogram by printf() function. Now you have to use the scanf() function to take input from the user and store in “base”, “height”, “length” and “width” variables respectively.
Next, calculate the area and perimeter of the parallelogram and store the result in “area”, and “perimeter” variables. Lastly, display the results on the screen by the printf() function. You can copy the below code of calculating the area and perimeter of parallelogram by C programming language and run on your pc.
/* Developd by Puskar Jasu*/
#include <stdio.h>
int main()
{
float base, height, length, width, area, perimeter;
printf("Enter the base of the parallelogram: ");
scanf("%f", &base);
printf("Enter the height of the parallelogram: ");
scanf("%f", &height);
printf("Enter the length of the parallelogram: ");
scanf("%f", &length);
printf("Enter the width of the parallelogram: ");
scanf("%f", &width);
area = base * height;
perimeter = 2 * (length + width);
printf("Area of the parallelogram = %.2f ", area);
printf("\nPerimeter of the parallelogram = %.2f ", perimeter);
return 0;
}
Output:
After running the above code you can see the output of calculating the area and perimeter of parallelogram by C programming language.
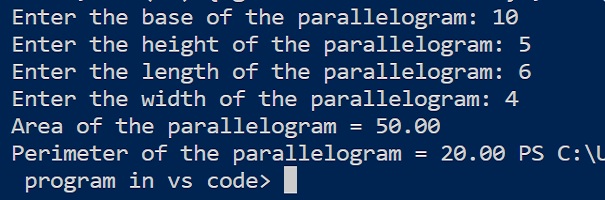
Calculate the area and perimeter of rhombus by C programming language :
In this program, I shall show you how to calculate the area and perimeter of a rhombus using the C programming language. Quadrilateral with all sides of equal length is called rhombus. To calculate the area and perimeter of a rhombus, you need to know the length of its sides and diagonals.
The area of a rhombus can be calculated by multiplying the diagonals of the rhombus and dividing the result by 2. The formula for area of a rhombus is given below.
Area = (diagonal1 x diagonal2) / 2
The perimeter of a rhombus is the sum of the lengths of all four sides. The formula of perimeter is given below.
Perimeter = 4 x side
Where diagonal1 and diagonal2 are the lengths of the two diagonals and side is the length of one of the sides of the rhombus.
explanation of the program :
Firstly, you have declared the required float variables for “diagonal1”, “diagonal2”, “side”, “area”, and “perimeter”. Then, you have used the printf() function for asking the user for entering the value of diagonal1, diagonal2 and side. Then use scan() function to take input from the user and store in “diagonal1”, “diagonal2” and “side” variables.
After that calculate the area and perimeter of the rhombus and the result store in “area” and “perimeter” variables. Finally, using printf() function prints the results on screen. You can copy the below code of calculating the area and perimeter of rhombus by C programming language and run on your pc.
/* Developd by Puskar Jasu*/
#include <stdio.h>
int main()
{
float diagonal1, diagonal2, side, area, perimeter;
printf("Enter the length of diagonal 1: ");
scanf("%f", &diagonal1);
printf("Enter the length of diagonal 2: ");
scanf("%f", &diagonal2);
printf("Enter the length of a side: ");
scanf("%f", &side);
area = (diagonal1 * diagonal2) / 2;
perimeter = 4 * side;
printf("Area of the rhombus = %.2f ", area);
printf("\nPerimeter of the rhombus = %.2f ", perimeter);
return 0;
}
Output:
After running the above code you can see the output of calculating the area and perimeter of rhombus by C programming language.

Calculate the area and perimeter of trapezium by C programming language :
In this program, I shall show you how to calculate the area and perimeter of a trapezium using the C programming language. The quadrilateral with one pair of parallel sides is called trapezium. Calculating the area and perimeter of a trapezium, you have to know the lengths of its parallel sides and its height.
The area of a trapezium calculates by multiplying the sum of the lengths of the parallel sides by the height between them and dividing the result by 2. The formula for calculating the area of a trapezium is given below.
Area = (sum of parallel sides) x height / 2
The perimeter of a trapezium is the sum of the lengths of all four sides. The formula for calculating the perimeter of a trapezium is given below.
Perimeter = sum of all sides
explanation of the program :
In the program, first you have to declare the required float variables such as “parallel_side1”, “parallel_side2”, “non_parallel_side1”, “non_parallel_side2”, “height”, “area”, and “perimeter”. Then, you have used the printf() and scan() functions to take input from the user for all the required sides and height.
After that calculate the area and perimeter of the trapezium and store in “area” and “perimeter” variable respectively. Finally, display the results using the printf() function in the screen. You can copy the below code of calculating the area and perimeter of trapezium in C programming language and run on your pc.
/* Developd by Puskar Jasu*/
#include <stdio.h>
int main()
{
float parallel_side1, parallel_side2, non_parallel_side1, non_parallel_side2, height, area, perimeter;
printf("Enter the length of parallel side 1: ");
scanf("%f", ¶llel_side1);
printf("Enter the length of parallel side 2: ");
scanf("%f", ¶llel_side2);
printf("Enter the length of non-parallel side 1: ");
scanf("%f", &non_parallel_side1);
printf("Enter the length of non-parallel side 2: ");
scanf("%f", &non_parallel_side2);
printf("Enter the perpendicular distance between the parallel sides: ");
scanf("%f", &height);
area = ((parallel_side1 + parallel_side2) * height) / 2;
perimeter = parallel_side1 + parallel_side2 + non_parallel_side1 + non_parallel_side2;
printf("Area of the trapezium = %.2f ", area);
printf("\nPerimeter of the trapezium = %.2f ", perimeter);
return 0;
}
Output :
After run the above code you can see the below output.

Calculate the area and perimeter of equilateral triangle by C programming language :
In this program, I shall show you how to calculate the area and perimeter of a equilateral triangle using the C programming language. A triangle with three equal sides and three equal angles of 60 degrees each called equilateral triangle.
To calculate the area of an equilateral triangle, you can use the following formula.
area = (√3/4) x (side)2
To calculate the perimeter of an equilateral triangle, just multiply the length of one side by three.
perimeter = 3 x side
Where side is the length of one side of the equilateral triangle.
explanation of the program :
In this program, first declare three float variables such as “side”, “perimeter” and “area”. Using the printf() function ask the user to enter the lengths of the side of the equilateral triangle. By the scanf() function, store the length of the triangle in “side” variable.
Next, calculate the perimeter and area of the equilateral triangle and store in “perimeter” and “area” variables. After that using the printf() function display the calculated area and perimeter of the equilateral triangle to the screen. You can copy the below code and use in your program.
/* Developd by Puskar Jasu*/
#include <stdio.h>
#include <math.h>
int main()
{
float side, area, perimeter;
printf("Enter the length of the side of the equilateral triangle: ");
scanf("%f", &side);
perimeter = 3 * side;
area = (sqrt(3)/4) * pow(side, 2);
printf("Area of the equilateral triangle = %.2f\n", area);
printf("Perimeter of the equilateral triangle = %.2f\n", perimeter);
return 0;
}
Output :
After run the above code you can see the below output.

Calculate the area and perimeter of right-angled triangle by C programming language :
In this program, I shall show you how to calculate the area and perimeter of a right-angled triangle using the C programming language. A triangle with one right angle (90 degrees) is called right-angled triangle.
The opposite side of the right angle is called the hypotenuse and the other two sides are called the legs.
The following formula is used for calculating the area of a right-angled triangle.
Area = (1/2) x base x height
Where the base and height are the two legs of the triangle.
The formula for the perimeter of a right-angled triangle is shown in below.
Perimeter = base + height + hypotenuse
Where the hypotenuse is calculated by the following formula.
hypotenuse = √(base2 + height2)
explanation of the program :
In the program, first declare five float variables such as “base”, “height”, “hypotenuse”, “area” and “perimeter”. Then ask the user to enter the base and height of the right-angled triangle by printf() function and using scanf() function, which store in “base” and “height” variables respectively.
After calculating the area and perimeter of the right-angled triangle and store in the “area” and “perimeter” variables respectively. Finally, you can use the printf() function to display the area and perimeter of the right-angled triangle on the screen. You can copy the below code and use in your program.
/* Developd by Puskar Jasu*/
#include <stdio.h>
#include <math.h>
int main() {
float base, height, hypotenuse, area, perimeter;
printf("Enter the length of the base of the right-angled triangle: ");
scanf("%f", &base);
printf("Enter the length of the height of the right-angled triangle: ");
scanf("%f", &height);
hypotenuse = sqrt(base * base + height * height);
perimeter = base + height + hypotenuse;
area = (base * height)/2;
printf("Area of the right-angled triangle = %.2f\n", area);
printf("Perimeter of the right-angled triangle = %.2f\n", perimeter);
return 0;
}
Output :
After run the above code you can see the below output.
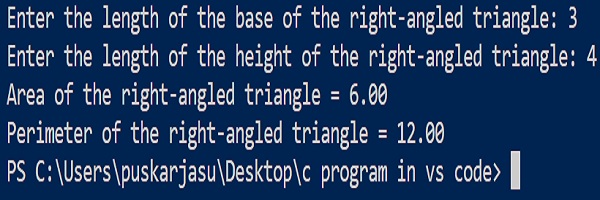
Calculate the area and perimeter of isosceles triangle by C programming language :
In this program, I shall show you how to calculate the area and perimeter of an isosceles triangle using the C programming language. An isosceles triangle is a triangle in which two sides have the same length. The third side is called the base.
The formula for the area of an isosceles triangle is given below.
Area = (1/2) x base x height
The formula for the perimeter of an isosceles triangle is given below.
Perimeter = 2 x side + base
Where the side is the length of the two equal sides and the base is the length of the third side and height is the perpendicular distance from the base to the opposite angle.
explanation of the program :
In this program, first declare five float variables such as “base”, “side”, “height”, “perimeter” and “area”. Using the printf() function ask the user to enter the lengths of base, side and height of the isosceles triangle. By the scanf() function, store the length of the triangle in “base”, “side” and “height” variables respectively.
Next, calculate the perimeter and area of the isosceles triangle and store in “perimeter” and “area” variables respectively. After that using the printf() function display the calculated area and perimeter of the isosceles triangle to the screen. You can copy the below code and use in your program.
/* Developd by Puskar Jasu*/
#include <stdio.h>
int main()
{
float base, side, height, area, perimeter;
printf("Enter the length of the base of the isosceles triangle: ");
scanf("%f", &base);
printf("Enter the length of the equal side of the isosceles triangle: ");
scanf("%f", &side);
printf("Enter the length of the height from the base to the opposite angle: ");
scanf("%f", &height);
perimeter = 2 * side + base;
area = (base * height) / 2;
printf("Area of the isosceles triangle = %.2f\n", area);
printf("Perimeter of the isosceles triangle = %.2f\n", perimeter);
return 0;
}
Output :
After run the above code you can see the below output.

Conclusion :
Calculating the area and perimeter of geometrical shapes is very popular and important operation in many areas of mathematics, science, and engineering. Using C programming language, you can easily calculate the area and perimeter of different geometrical shapes.
In this article, I explain the basic concepts of area and perimeter and give various codes of programs which show the calculations for each geometrical shape. After understanding these formulas and program you can efficiently solve real-world problems related to geometry.
Thank you for visiting my site.