Introduction :
In programming, random number generation is a fundamental task in various computer programs. It is used in many software applications such as game development, cryptography program, statistical analysis and more. In C programming language, you can use the rand() function for generating pseudo-random numbers. Using rand() function, you can add randomness to your C applications or projects.
To generate pseudo-random numbers is the basic usage of the rand() function in C programming language. In this article, I shall show you how to use rand() function using the C programming language. Here, I also show you the syntax and usage of rand() function.
What is rand() function :
The rand() function is a function of the standard library file (stdlib.h) in C programming language. Using the rand() function, you can generate random numbers (pseudo) in C programming language. By default, the rand() function returns a pseudo-random integer in the range 0 to RAND_MAX. The RAND_MAX is a constant which is defined in stdlib.h library or header file. If you want to generate numbers within a specific range, you have to use the modulo operator (%) to limit the range.
The stdlib.h library contains the declaration of rand() function, so you have to include this library at the beginning of your program. If you want to generate a different random number each time you run your program, you have to seed the random number generator using the srand() function. You can seed the random number generator with the current time.
Syntax of the rand() function :
The following code is the syntax of the rand() function in C programming language.
int rand(void);
The rand() function does not take any parameter or argument and it returns an integer value between 0 and RAND_MAX.
About the program :
When you run the program on your pc, you can see various random numbers (int and float) and characters are displayed on the screen. Here, you see random integer number, random integer number from 50 to 100, different type of character and floating number from 0 to 1 on the console screen. Every time you run the program, the numbers and character will be changed.
Explanation of the program :
First, you need to include stdio.h, stdlib.h and time.h library in your program. In the main() function, you can declare integer variables “random_int”, “random_int_range”, “min_int” and “max_int”. Then declare char and float variables such as “random_char” and “random_fraction_number” respectively. Now, you can seed the random number generator using the current time with srand() function.
After that, initialize the random int, char and float value in different variables with rand() function. Using printf() function, you can display the generated random numbers (int and float) and characters on the console screen.
How run the program :
To run the program, you have to install VS Code on your pc. If you want to run other C complier like Turbo C++ IDE, install Turbo C++ IDE on your pc. After install VS Code, open it and create new file give name as “pac.c”. Then copy the below code and paste in the “pac.c” file. Now, save the file and run on your pc.
Source code of the program :
The following code is used for rand() function in C programming language.
/*Developed by Puskar Jasu*/
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main()
{
int random_int, random_int_range, min_int = 50, max_int = 100;
char random_char;
float random_fraction_number;
srand(time(NULL));
random_int = rand();
random_int_range = rand() % (max_int - min_int + 1) + min_int;
random_char = rand() % 128;
random_fraction_number = (float)rand() / RAND_MAX;
printf("Random integer number --- %d\n", random_int);
printf("Random number between %d and %d is %d\n", min_int, max_int, random_int_range);
printf("Random chatacter --- %c\n", random_char);
printf("Random fraction number --- %f \n", random_fraction_number);
return 0;
}
Output of the program :
After running the program on your pc, you can see the output of the program like below image.
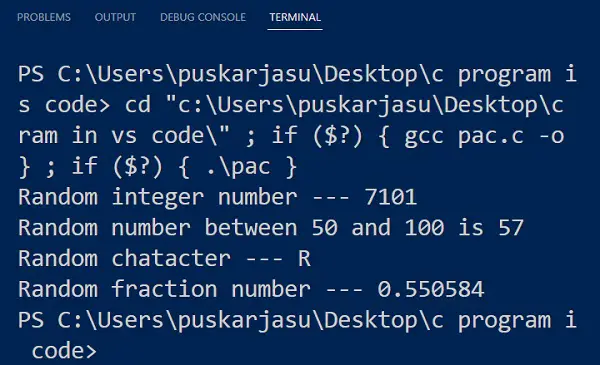
Conclusion :
Finally, you have learned how to use rand() function in the C programming language. Now, you can use rand() function to create more complex programs as you like. Thank you for visiting my site.