Introduction :
In C programming language, characters are represented by their individual ASCII values. The ASCII value is a unique integer value of a character, which store in char variable rather than that character itself. In this article, I shall show you how to display ASCII value of character in C programming language.
What is ASCII value :
American Standard Code for Information Interchange is generally known as ASCII. ASCII is a character encoding system that is used for electronic communication. There are 256 characters (0 to 255) use in a computer system, but only first 128 characters (0 to 127) are called ASCII characters.
These include lowercase letters, uppercase letters, digits, special symbols, etc. Remaining 128 characters (128 to 255) are called Extended ASCII character or graphics characters. The graphics characters are used to draw single or double line and boxes in text mode.
Different types of program with ASCII value :
In this article, I shall discuss with you the following programs in C programming language. First, you have to learn how to run the all programs on your PC. In this article, I use VS Code to run the all programs. If you do not know how install VS Code on your PC, just click this link. Now open VS Code, create a C file with .c extension. Then, copy the below code and paste it in the C file. To see the output of the program, run it.
Find the ASCII value of a character in C programming language :
In this program, I show you how you print the ASCII value of a character in C programming language. First, you have to declare a character (char) variable “ch”. It will be stored the character whose ASCII value you want to print. Then, ask the user to enter a character using printf() function. Now, store the input in “ch” variable using scanf() function.
After that, using printf() function, you can display the ASCII value and the character on the console screen. Here, I use the “%d” format specifier to print ASCII value and “%c” format specifier to print actual character in the printf() statement. You can copy the below code and run it to see the output of the program.
/* Developd by Puskar Jasu*/
#include <stdio.h>
int main()
{
char ch;
printf("Enter a character for print its ASCII value\n");
scanf("%c", &ch);
printf("ASCII value of %c is %d\n", ch, ch);
return 0;
}
Output :
You see the below image as output to find the ASCII value of a character in C programming language.
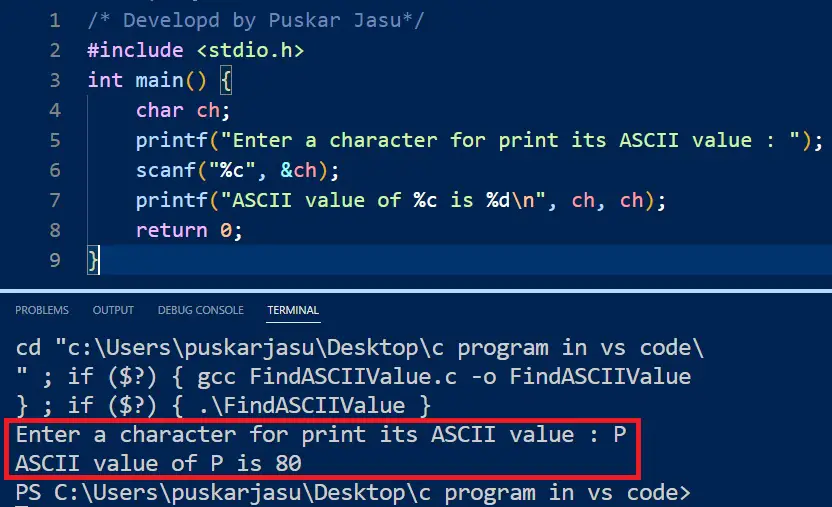
Display the character input by ASCII value(Integer) in C programming language :
In this program, I show you how to print the character input by ASCII value or Integer from 0 to 255 in C programming language. First, you have to declare an integer(int) variable “num” in the program. It will store the integer number by which you print the character. Then, ask the user to enter a number from 0 to 255 using printf() function.
Then, store the input in “num” variable using scanf() function. Now, using printf() function you can print the character with ASCII value. Here, use the “%c” format specifier to print the character and “%d” format specifier to print actual integer in the printf() statement. Now, copy the below code and paste it in C file and run it to see the output.
/* Developd by Puskar Jasu*/
#include <stdio.h>
int main()
{
int num;
printf("Enter a number from 0 to 255 for print its character\n");
scanf("%d", &num);
printf("The character of %d integer is %c\n", num, num);
return 0;
}
Output :
You can see the below image as output of display the character input by ASCII value (Integer) in C programming language.

Display all the characters with the ASCII value in C programming language :
In the program, I show you how to print all the characters with the ASCII value in C programming language. Here, I use a for loop to print all the 256 characters with their corresponding ASCII values. You can use “%c” and “%d” format specifiers in printf() function. There are NULL, BS (backspace), STX (Start of Text) etc. include among the first 31 ASCII characters.
When you print them, these characters do not display properly in the console. Now, copy the below code and paste it in C file and run it to see the output on the console.
/* Developd by Puskar Jasu*/
#include <stdio.h>
int main()
{
for (int i = 0; i <= 255; i++)
{
printf("The ASCII value of %c is %d \n", i, i);
}
return 0;
}
Output :
You see the below image as output of display all the characters with the ASCII value in C programming language.
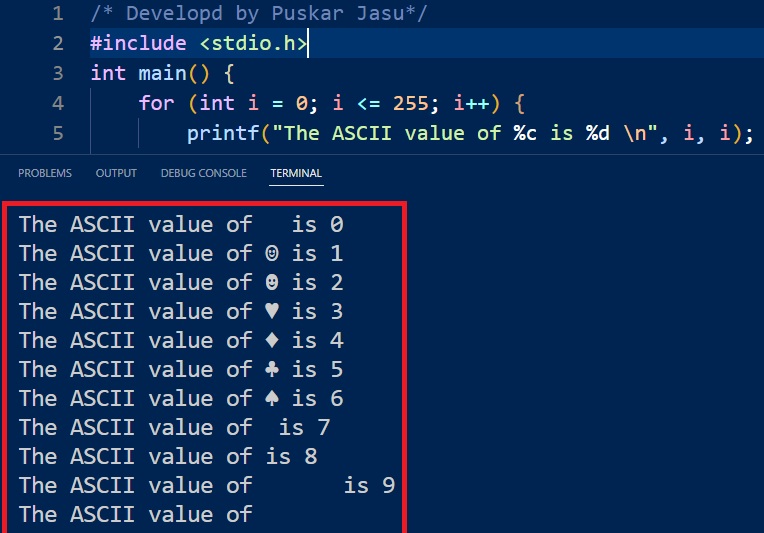
Display all the alphabets with the ASCII value in C programming language :
In the program, I show you how to print all the alphabets with the ASCII value in C programming language. You can use a for loop to print all the 26 Capital and Small case alphabets with their corresponding ASCII values. Here, I use a condition in “if” statement. The capital letters lie between 65 to 90 and small case letters lie between 97 to 122.
Using condition in “if” statement, I filter all the letters and print them on the screen. Now, copy the below code and paste it in C file and run it to see the output.
/* Developd by Puskar Jasu*/
#include <stdio.h>
int main()
{
for (int i = 0; i <= 255; i++)
{
if ((i >= 65 && i <= 90) || (i >= 97 && i <= 122))
{
printf("The ASCII value of %c is %d \n", i, i);
}
}
return 0;
}
Output :
You see the below image as output of display all the alphabets with the ASCII value in C programming language.
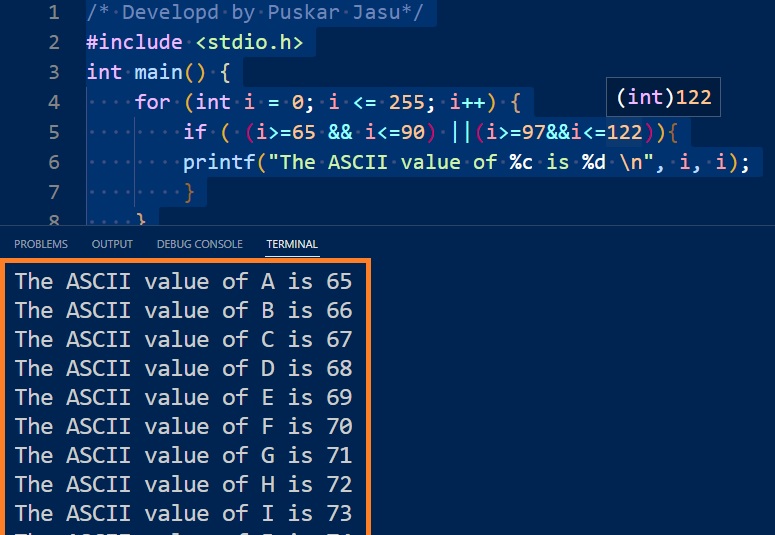
Conclusion :
In above article, I have discussed with you about how to find the ASCII value of a character, display the character input by ASCII value or integer, display all the characters with the ASCII value and display all the alphabets with the ASCII value in the C programming language. I think you understood how all the programs run. Thanks you for visiting my site.